Using BRO for mass-creation of materials
Since I am an ABAP developer and I am also using BRO since 2017 - I want to show off a great capability of BRO: easily creating many materials in SAP at once with arbitrary data. The cool parts:
- It can use calculation logics. This makes it way more powerful then the transaction MM17 - which only takes the final values for the database tables of a material.
- No ABAP logic is necessary.
But first off: what is BRO? BRO stands for Business Rules Organizer and is a paid SAP ERP addon allowing creating business rules. The rules themselves are organized in a completely different way than its direct competitor BRF+ (Business Rules Framework+) which is an official module within SAP ERP. The organization of rules is actually really unique. A rule consists of steps which itself consists of conditions and actions. The unique part is that the actions:
- allow calling other rules which are then in turn executed.
- allow calling function modules and thus arbitrary Z-code.
- are using action types which allow custom implementations in ABAP too.
tl;dr a BRO rule is a subroutine containing a big IF-ELSE.
This features allows a seamless switch between actual business rules (containing only data) and low-level stuff like saving a material.
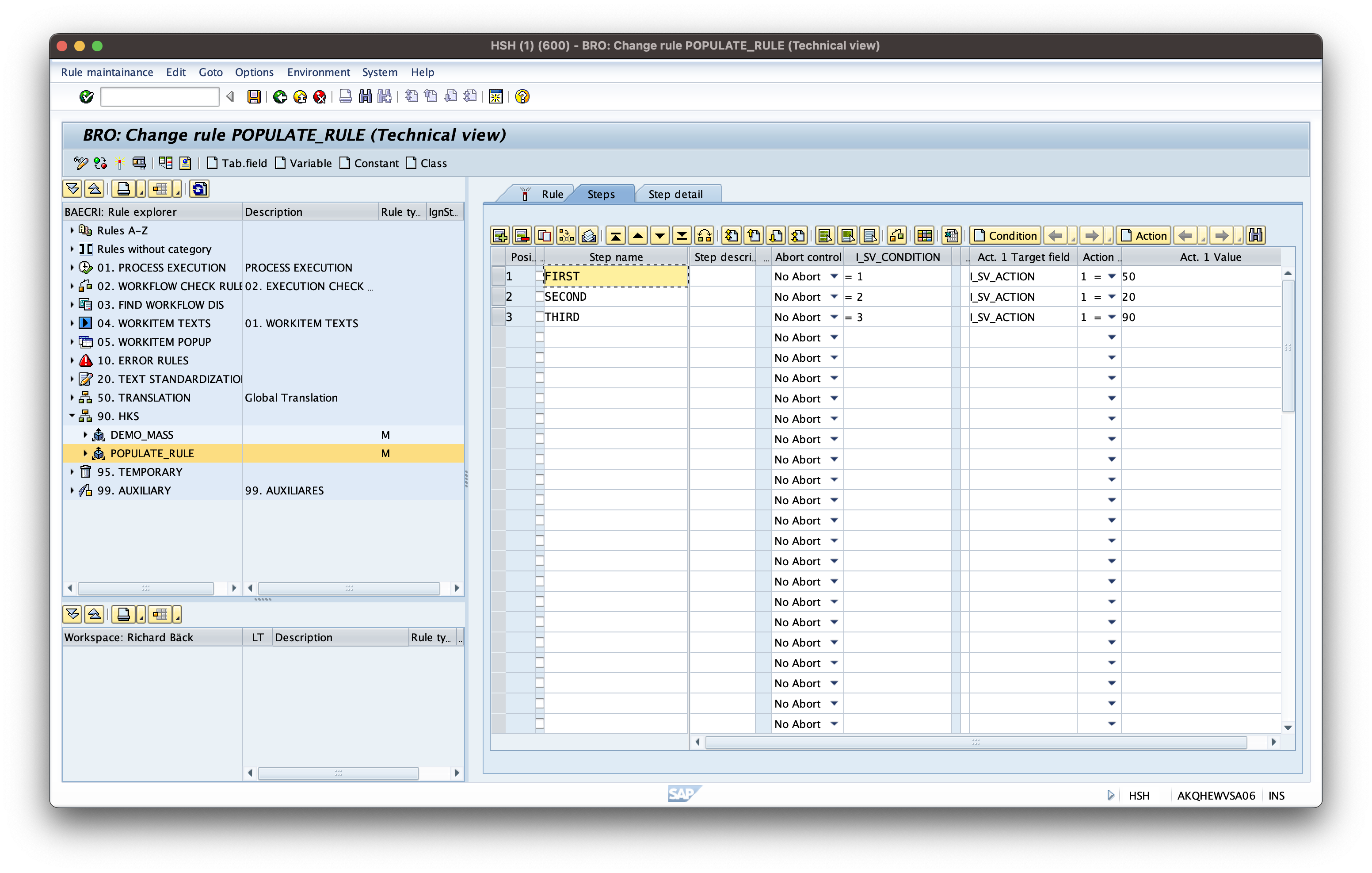
A rule with some conditions and actions
Prerequisites
- Basic knowledge on BRO.
- Since you are most likely a BRO user already, please make sure that you are running at least BRO 5.4.3 with its default customizing.
- You are running the latest material low-level rules of the bRobots MDM+ Material decision area that are available for BRO 5.4.3.
- To check this, check if the rule BMAP_BAPIE1MARA_MAPPED is available.
- Basic knowledge on the database tables for materials in SAP. E.g. MARA, MARC
The goal
The goal is to easily create multiple commodities at once with only a minimum of basic data and a single material description in English.
Setup
Creating the rule
The start rule will be one material per step. This will allow us to have an easy view on the materials to be created. We can create the new rule by simply doing the following:
- Right click on the category 90. (The category might be named with the name of your company) and click Create new rule
- Use the name DEMO_MASS for the new rule and hit Enter.
- Rule names are not case sensitive and will always be converted to upper case.
- Rule names are not case sensitive and will always be converted to upper case.
- Now set the data on the rule header
- Use Multi-hit as the Rule type. This is necessary to execute all steps from top to bottom if their condition apply.
- Single-hit will exit after the first step has been executed.
- It is a good practise to indicate a user group responsible for the rule by setting an Authority group. In my case I can use ADMIN.
- Use Multi-hit as the Rule type. This is necessary to execute all steps from top to bottom if their condition apply.
Adding the boilerplate code
You are now sitting in front of a new empty rule. You may already be able to fill MARA fields. But doing so right away will not lead to any results. Setting MARA fields alone will not result in creating a material. Since BRO will only do what it is told to do nothing will be done other than setting some fields during the runtime execution.
To actually create a material some boilerplate code is needed. Some special function modules must be called in a sequence. The first one being a function module to actually perform the material creation. The second one to commit the material to the database. So we will need the following function modules:
- /HKS/BRO_MDM_AC_MATERIAL
- /HKS/BRO_MDM_AC_COMMIT_WORK
- For testing purposes we use /HKS/BRO_MDM_AC_ROLLBACK_WORK for now. This will undo the changes scheduled by /HKS/BRO_MDM_AC_MATERIAL instead of committing them to the database.
So our first version might look like the following:
But this will not work at all (again). Those function modules are not to be called directly. They are somewhat magic function modules which are collected and executed automatically in sequence. Such automatically executed function modules are called action function module.
So to actually get it up and working correctly we have to use a function module which consumes the action function modules and is really executed right away. This function module is called /HKS/BRO_MDM_PROCESS_ACTION_FM. Adding this one will result in the following rule:
Additionally I have added two new variables:
- SV_HKS_IMMEDIATE = C_YES
- This will register action function modules that are stated after this statement for the function module /HKS/BRO_MDM_PROCESS_ACTION_FM.
- SV_ADD_BRO_MSG = C_YES
- This will result in outputting any error and success messages created by the action function modules
First test run
It is already possible to successfully execute the rule. But it will not result in doing anything at all. It does not even try to create materials - which should make sense to you since we have not stated any material data yet.
The rule can be executed without any inputs. The rule will remain not relying on any inputs as everything needed by the main action function module /HKS/BRO_MDM_AC_MATERIAL will be stated within the rule anyway.
You may now execute the rule to check if it does not lead to a system dump. 😊
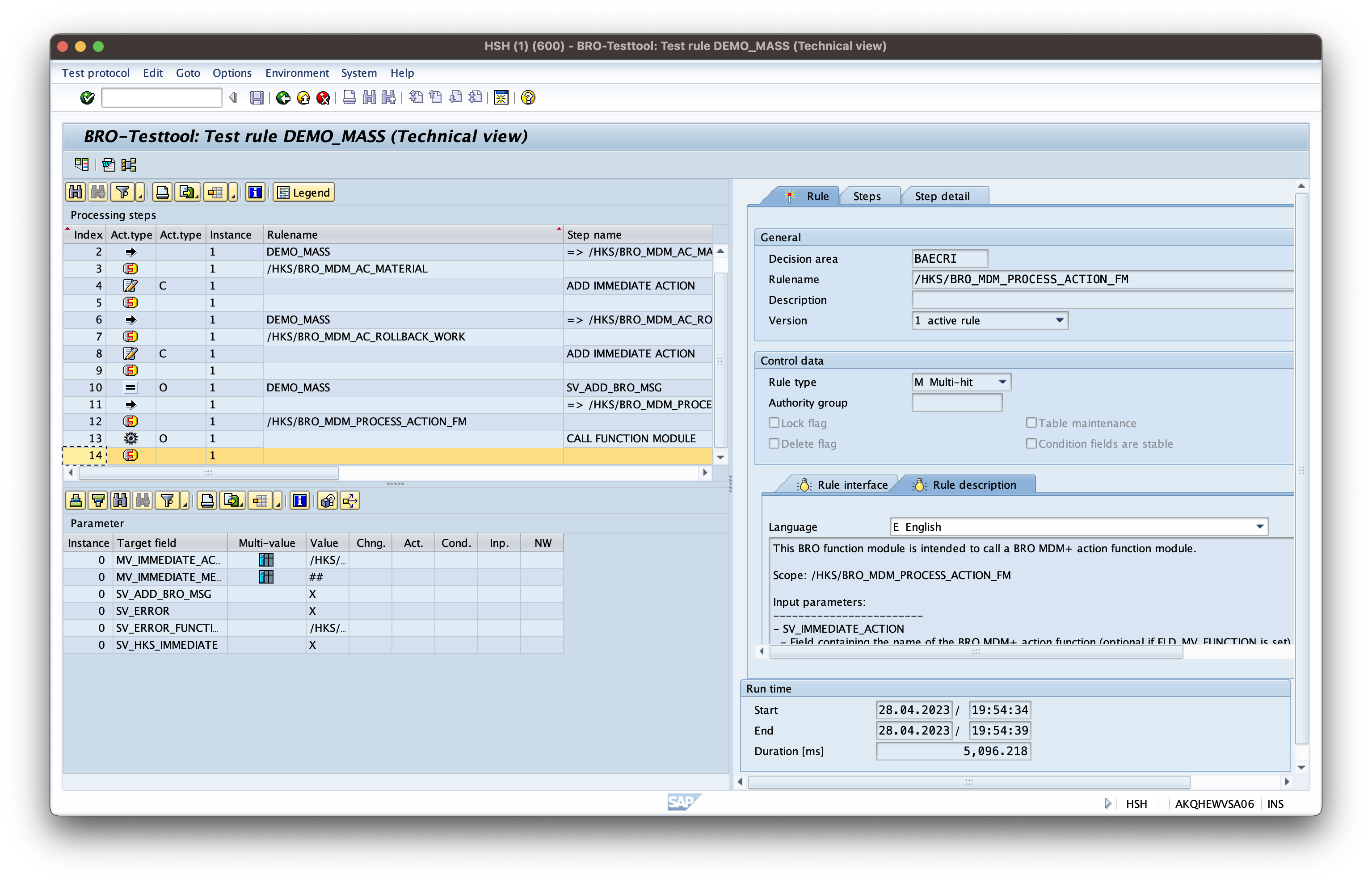
Results of the first test run - nothing at all
Adding the boilerplate code II
Before we can finally start stating material data we have to add some remaining boiler plate code. To explain why we are using the upcoming boilerplate code we have to first understand what the action function module /HKS/BRO_MDM_AC_MATERIAL is taking as inputs. Said action function module is using structures starting with BAPIE1 as inputs. The most important one is BAPIE1MARA which holds the - as you might already suspect - general data of the material. But please do not fall for the idea that this is straight forward. BAPIE1MARA is not the same as the database table MARA. The difference are the names of the attributes which are abbreviations in English (e.g. BAPIE1MARA-MATL_GROUP which is applied to MARA-MATKL). But there are also some more minor differences.
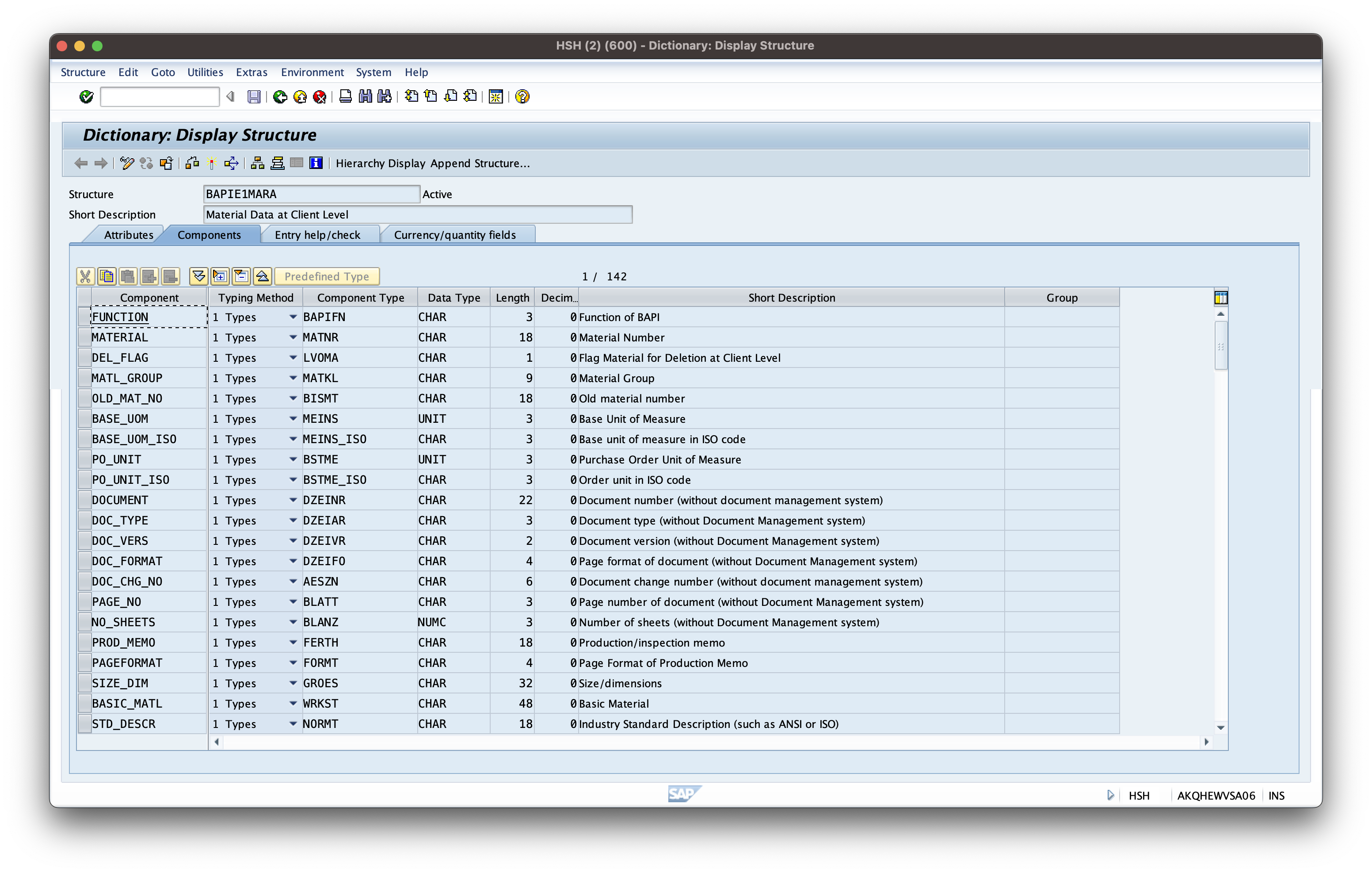
The first part of the structure BAPIE1MARA
This comes with the following down sides:
- Necessary fields cannot just be determined by using the technical view within the F1 help for a field in MM01/MM02/MM03
- The structure of the BAPI must be known and translated to what one want to actually achieve.
So to avoid those downsides we use an abstraction layer. This abstraction layer is called BAPI Mapping and is responsible for translating MARA fields to BAPIE1MARA fields and so on. BAPI Mapping is actually a 3 staged process:
- State once which fields should be updated (for a creation all fields should be updated). Stating to update all fields is as easy as calling the rule BMAP_BAPIE1MARA_BAPI_FIELDS.
- Move an entire MARA line into an internal buffer after the MARA line has been fully described. This is as easy as calling the rule BMAP_BAPIE1MARA_MAPPED.
- After the last MARA line has been moved, the entire buffer has been processed to make it available for the action function module /HKS/BRO_MDM_AC_MATERIAL. This is as easy as calling the rule BMAP_BAPIE1.
For the sake of simplicity I have only considered the database table MARA in the last enumeration. But you may just substitute the name MARA with the database table you want to describe (i.e. MARC). Just keep in mind to keep the order and that the 3rd step must be done only once. The 1st step can be done for each line individually but in most cases the same attributes of all lines have to be updated and thus the rule is called only once.
Now to finish this chapter we just place the rules BMAP_BAPIE1MARA_BAPI_FIELDS and BMAP_BAPIE1 at start of the rule.
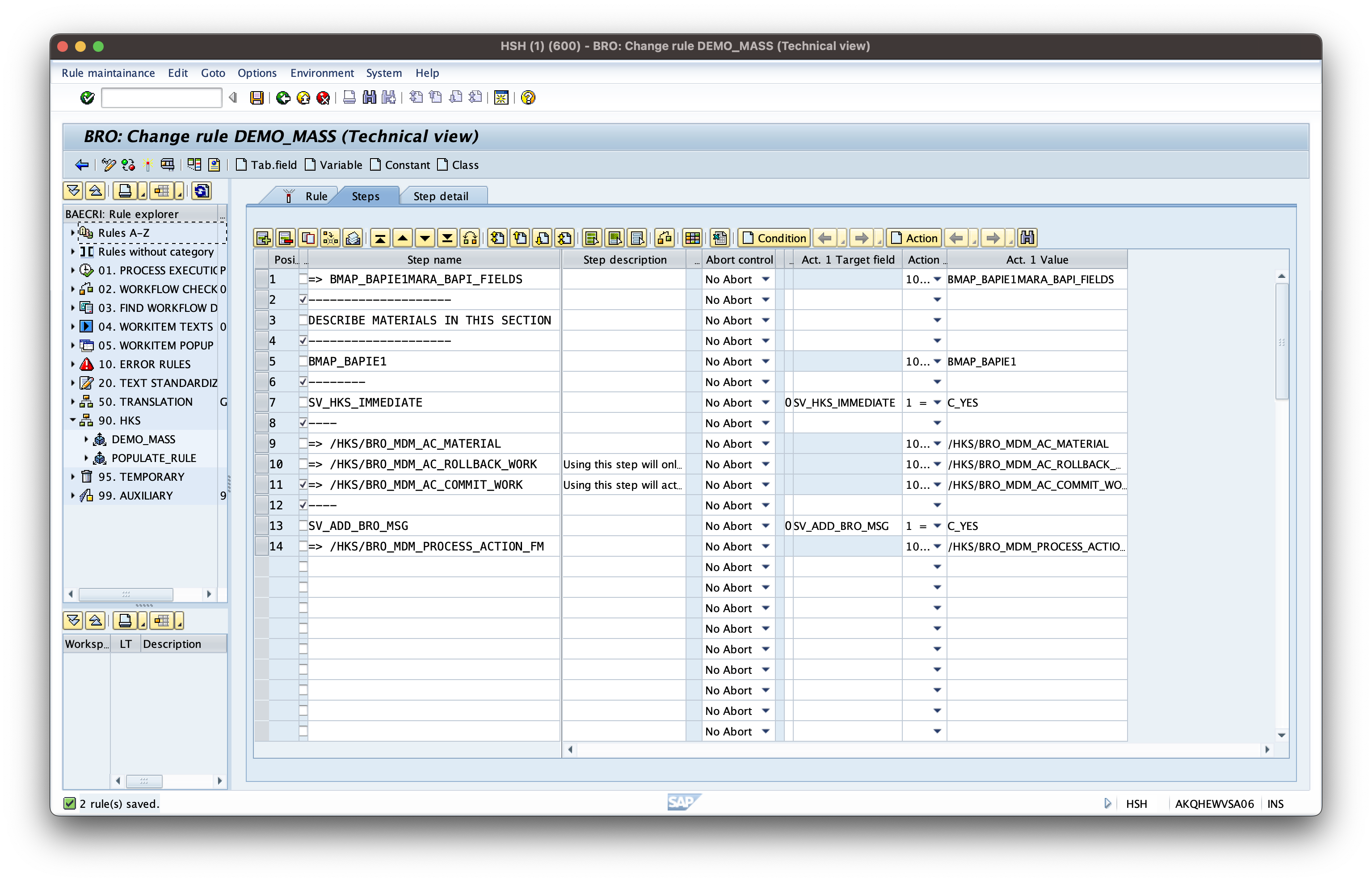
The rule with the remaining boilerplate code only
You may again execute the rule. But yet again nothing will happen.
Since we might add many materials it comes in quite helpful to outsource the description of the material fields in some other rule. Therefore we create a new rule called DEMO_MASS_DATA and place it between the new added steps.
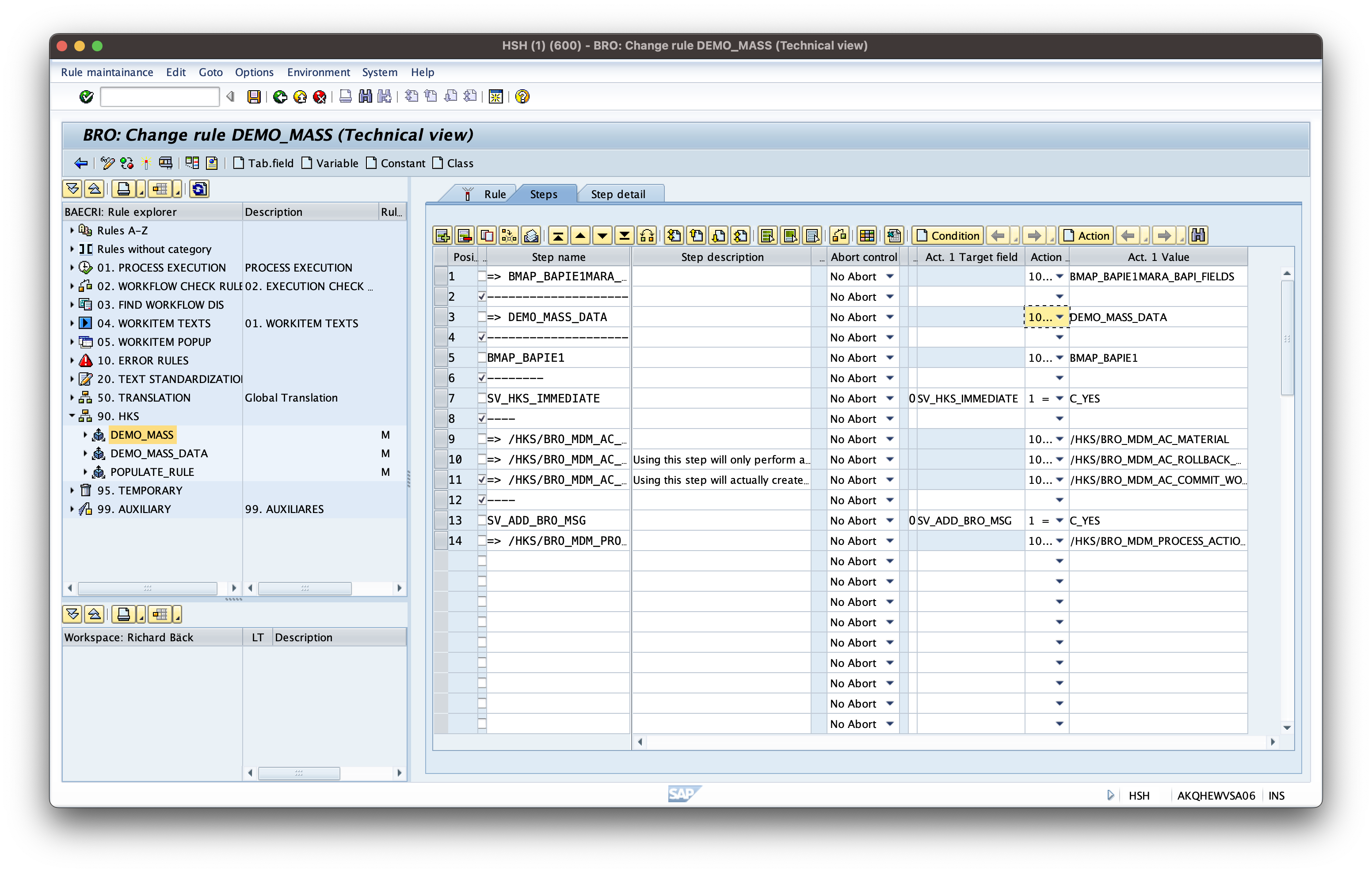
Updated main rule outsourcing the material data
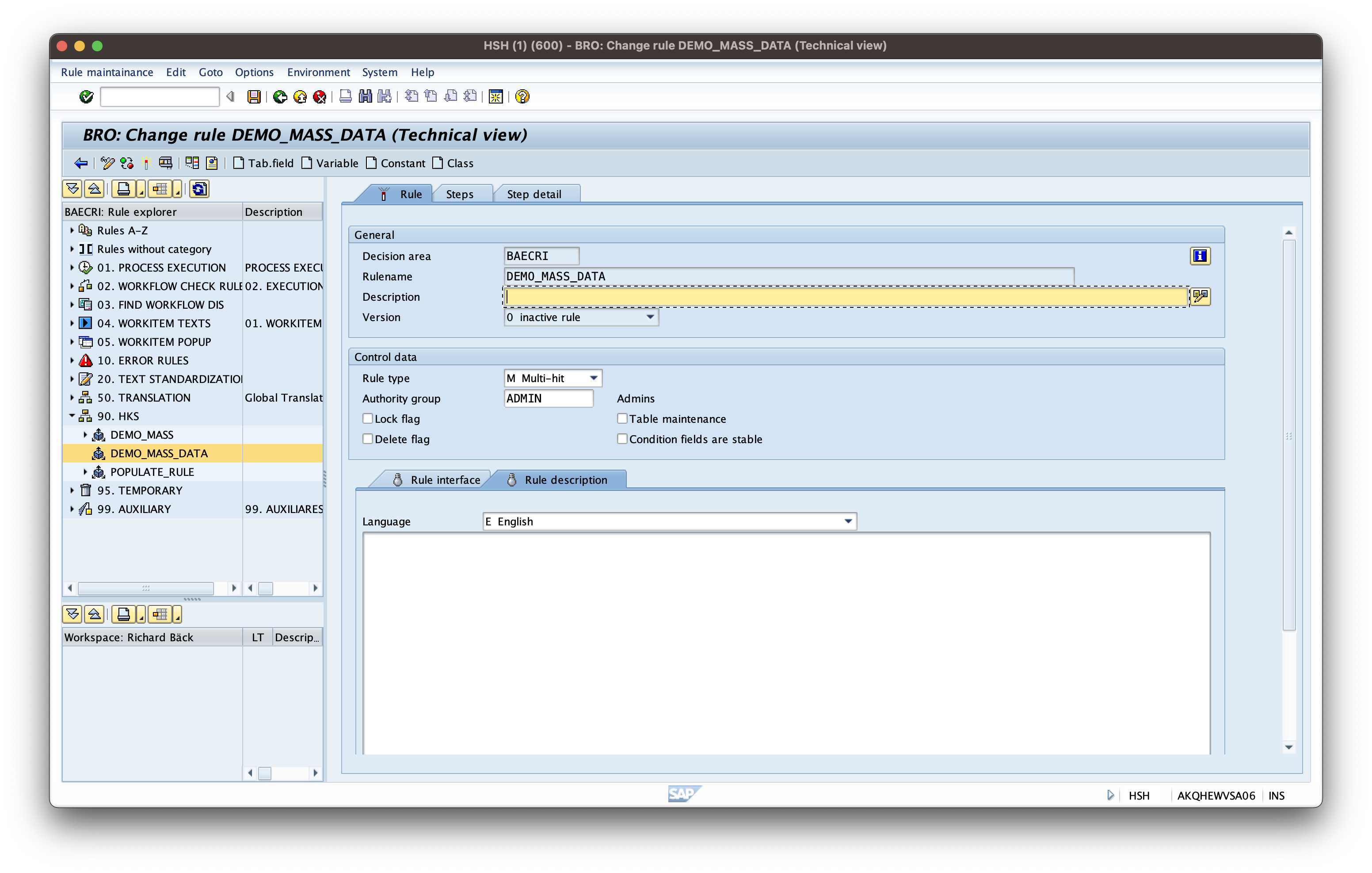
New rule carrying the actual material data to be created
Adding the data
The header line
Now finally we can add the data. But before we do so we think of a nice structure to ensure that the table is actually readable by fellow human beings. First we think of the attributes we need:
- The material number
- A must as otherwise we cannot identify the material
- For simplicity I expect you to use an external number range
- The industry sector
- A required field by the SAP standard
- The material type
- A required field by the SAP standard
- The material group
- A required field by the SAP standard
- The base unit of measurement
- A required field by the SAP standard
- The material description
- Not required by the SAP standard but it makes sense to state it
- The language of the material description
- Required by SAP as the material description is translatable
The material must supplied twice - unfortunately. A second time for the material description as the table MAKT also needs it.
Rotate this enumeration by 90 degrees and you have table headers. I place those table headers as comment line within the rule as reference into the rule. But you will see that you will not need that header at all later on.
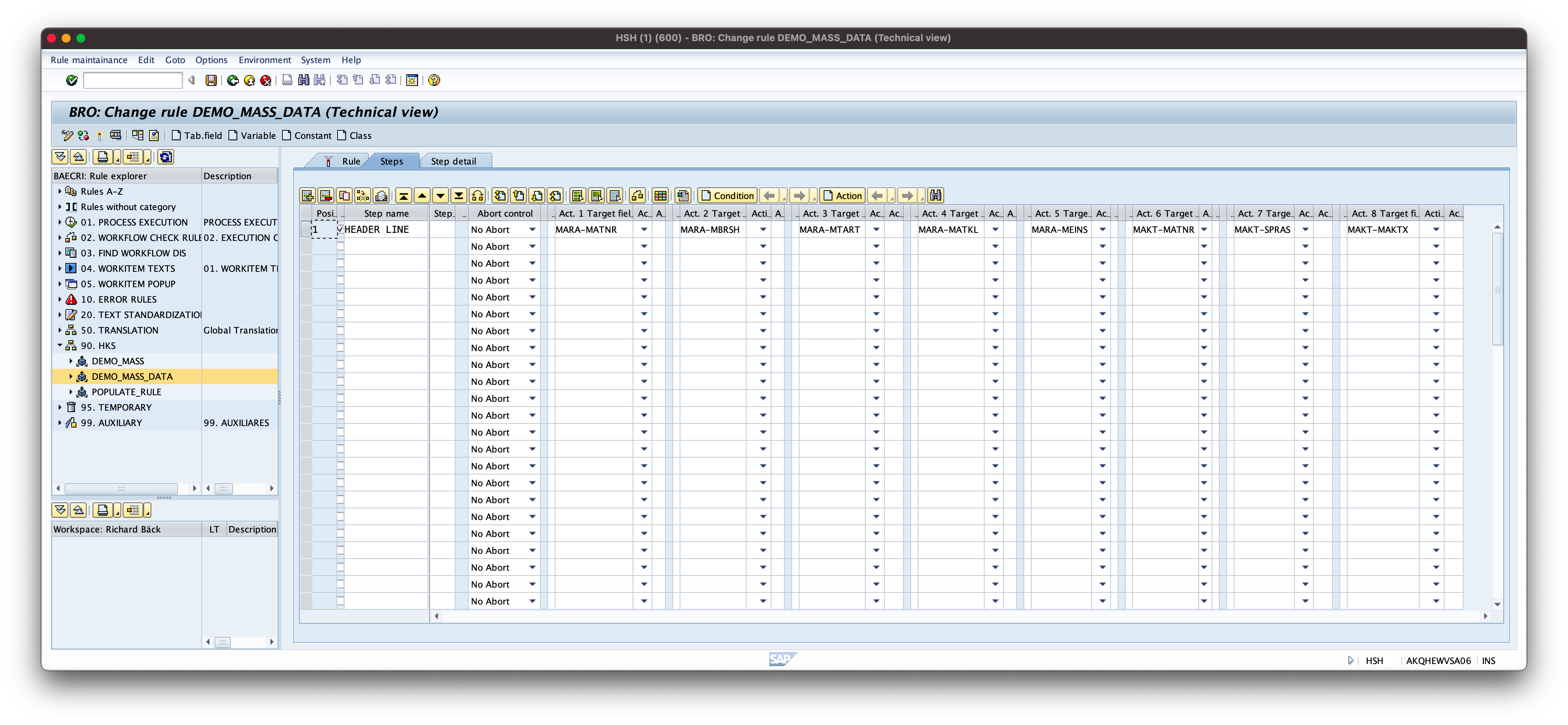
The data carrying rule with only the header line
The data
Alright, with this good looking header rule we can actually place some lines in there.
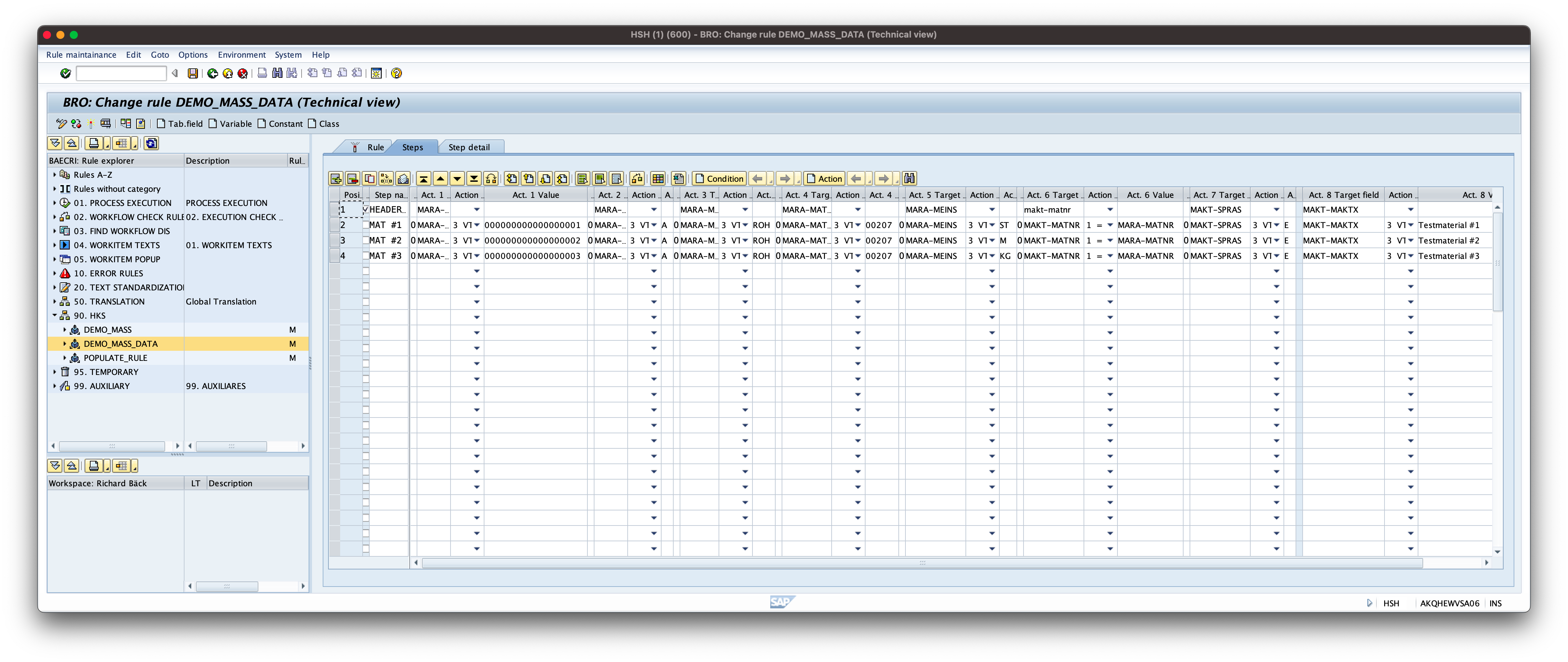
The data carrying rule with some data
On the data itself: it must be written fully in the internal SAP format. On the base unit of measurement: the cause for using ST instead of PC for piece is that SAP internally uses the value ST. This is also the same cause for supplying leading zeroes for the material number.
If you are a careful reader, then you might ask yourself now why I am not using the rule BMAP_BAPIE1MARA_MAPPED. The answer is: I just wanted to explain the basic idea without some weird looking functional rules this actually looks like a good ol’ Excel sheet.
So now we can place the following rule calls at the end of each line:
- BMAP_BAPIE1MATHEADER_MAPPED
- This is a special rule which is needed in tandem with BMAP_BAPIE1MARA_MAPPED to indicate which material views (in the sense of MM01) should be created.
- This rule will create the maximum available material views for the selected material type.
- BMAP_BAPIE1MARA_MAPPED
- BMAP_BAPIE1MAKT_MAPPED
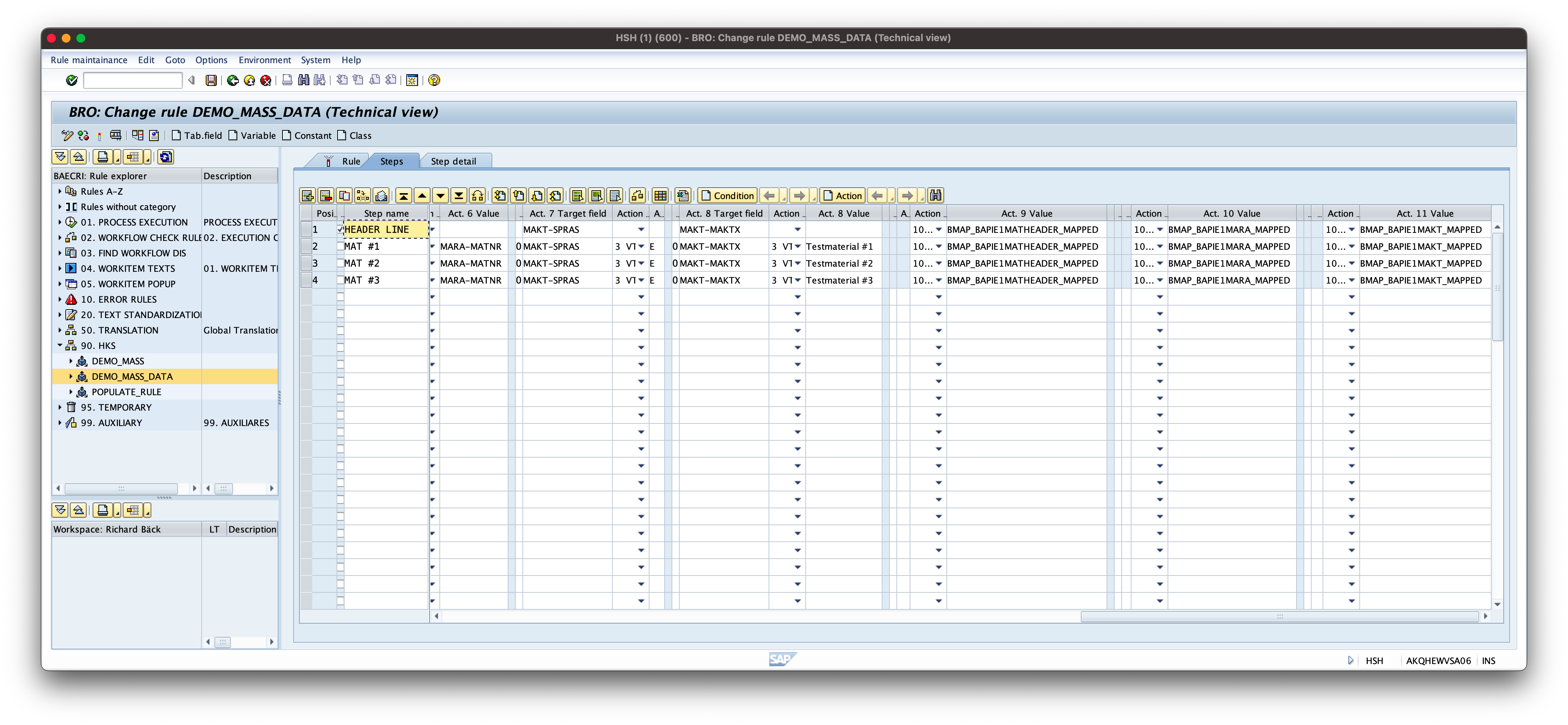
The data carrying rule fully functional
First actual test run
Now we can finally execute the rule DEMO_MASS and actually receive some meaningful outputs. To get the output messages you have to scroll down to the bottom in the table Processing steps and check the column Msg. changed. Double clicking its cell will present me the 3 success messages - one for each new material.
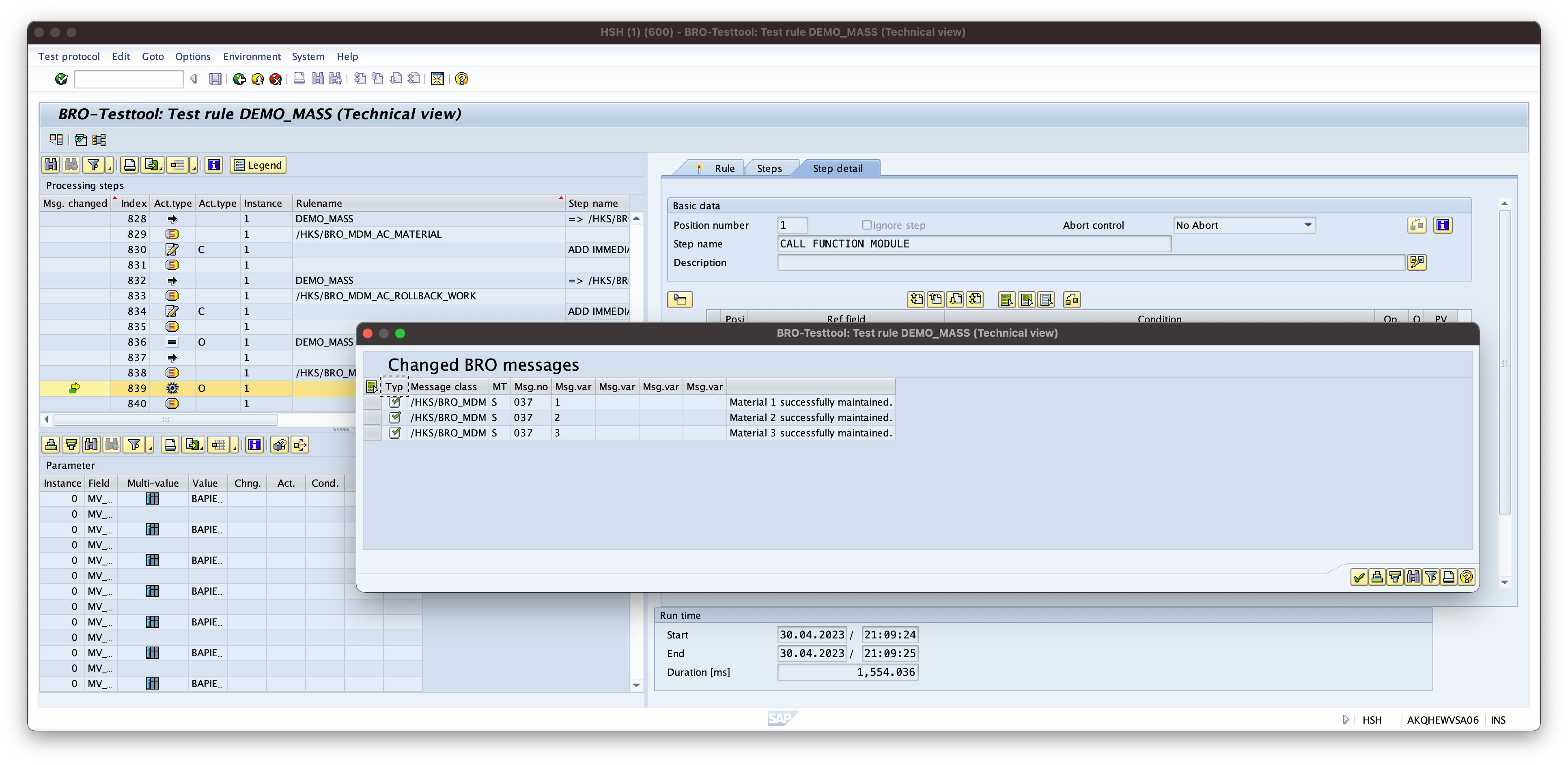
First actual test run creating materials
An important note: we are still rolling back the changes. So we can execute this rule as long as we want. Knowing this you can now play around with the data as much as you want. You can even explore further tables like the plant specific data (MARC) in which you are most likely interested.
Production run
To switch to a production run you have to simply swap the action function module /HKS/BRO_MDM_AC_ROLLBACK_WORK with /HKS/BRO_MDM_AC_COMMIT_WORK in the main rule. Running the rule afterwards will commit the created materials to the database and they are available in the transaction MM03 and so on immediately afterwards.
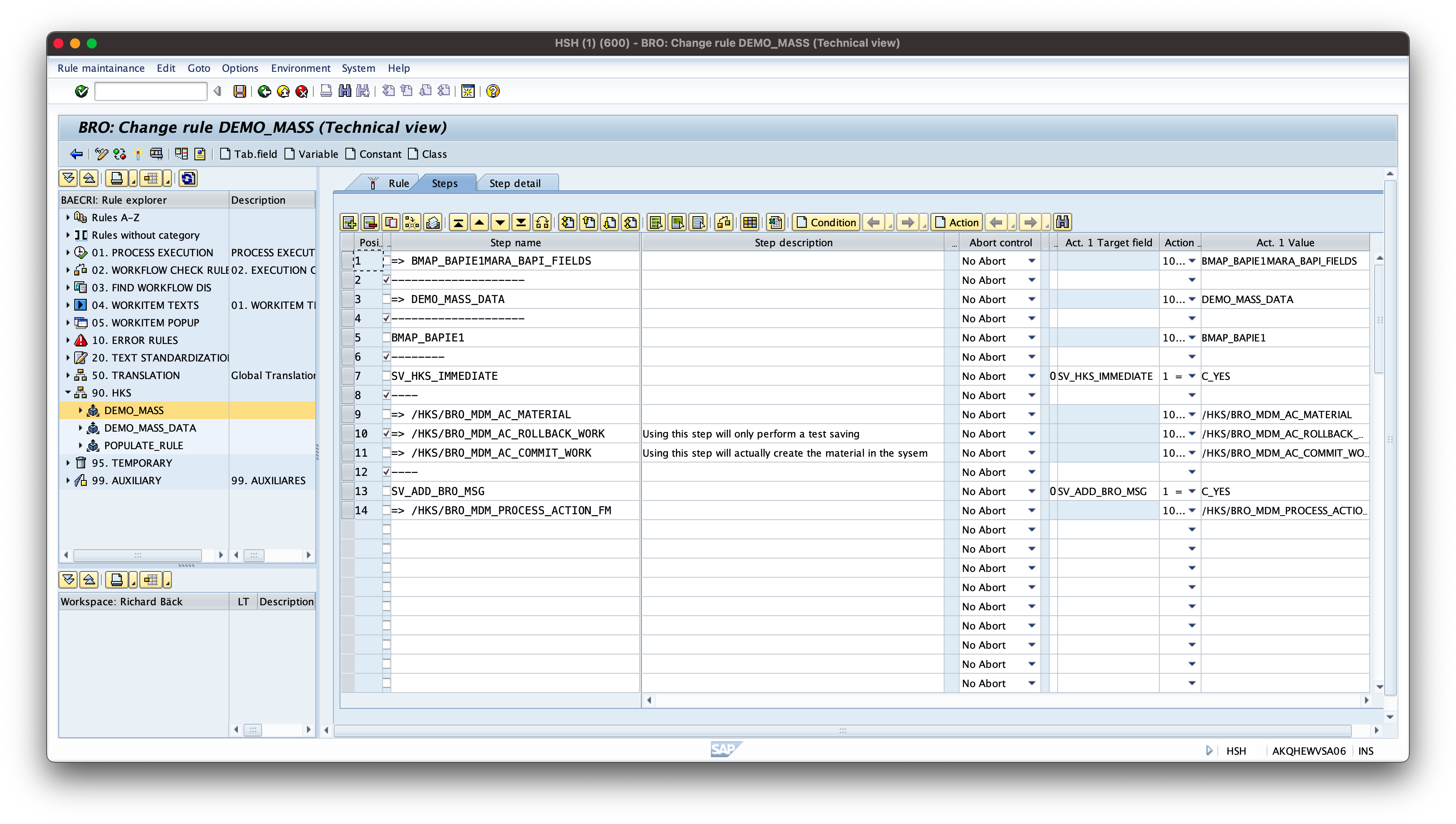
The main rule updated to be ready for a production run
Bonus: updating materials
Now that we know how to create materials - wouldn’t it be wonderful if we could update materials too? Especially if the production run was not tested well enough, this could come in very hand.
Luckily this is as easy as re-running the production run. Why? Because the action function module /HKS/BRO_MDM_AC_MATERIAL does not care whether the material exists or not. If it does not exist, then it is created. If it does exist, then it will just be updated.
So just make sure that you are not creating more materials then needed and you should be safe even with not well enough defined data.